C is a programming language, it is a method of communicating with a computer.
C is a particularly flexible language and has been used for micro-controllers, operating systems, applications and graphics programming. An improved C language called C++ has been invented and it does lots of things you don’t know about like calling constructors and destructors for variables. So C++ does some things automatically while C doesn’t.
You can’t speak english to a computer as there is too much ambiguity. However, there is a group of people who spend their time trying to write precise English. They’re called the government and the documents they write are called government regulations. unfortunately in their effort to make regulations precise the government has made them unreadable.
Machines use binary as their language. In order to give instructions (translate) to this language we use assembly language. Translation was tedious so a program was used for this task, called an assembler. The assembler made it easier for programmers to understand but harder for machines to use.
Thereafter a number of higher level languages were developed, such as FORTRAN, COBOL and PASCAL.
Brief History of C
In 1970, Dennis Richie, created a new language called C. The only goal of this programming language was to program operating systems. The language was simple and flexible and soon was used for many different types of programs. The language did not get in the way of the programmer, anything could be done using the proper C construct. The compiler was portable and widely available.
“C with Classes” was developed in 1980 and became C++, thereafter ‘C++ with the bugs fixed’ was called Java.
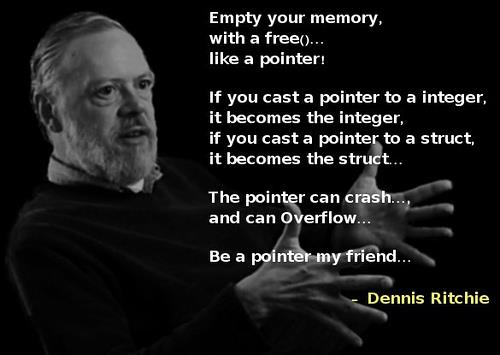
How C Works
C is a bridge between the programmer and the raw computer. The idea is to let the programmer organize a program in a way that can easily be understood. The compiler then translates the language into something that the machine can use.
Programs consist of data and instructions. The programmer should impose his organization on the computer.
Data is stored as a series of bytes and declarations are used to describe the information:
int total;
Tells the memory to store an integer named total.
int balance[100];
For more complex data types eg. a rectangle with a width, a height, a colour and a pattern.
struct rectangle{
int width;
int height;
color_type colour;
fill_type fill;
};
instructions:
area = (base * height) / 2.0;
An arithmetic assignment statement.
Standard control and selection statements such as if, switch, while and for are also present. Groups of reusable functions can be combined into a library, so you can borrow from the library with qsort for example.
All these structures are organised to benefit the programmer, not for the computer.
The source of this is not my own, it is from the book: Practical C Programming